概要
PHP 学習メモ。ファイル読み込み。
内容
- ファイル読み込み
- 検証
1. ファイル読み込み
ファイルを読み込むには include
と require
を使う。
上記ドキュメントで注意されているが、これらは関数ではなく文(statement)であり括弧で囲まないのが一般的らしい。
Remember, when using require that it is a statement, not a function. It's not necessary to write:
<?php require('somefile.php'); ?>The following:
<?php require 'somefile.php'; ?>Is preferred, it will prevent your peers from giving you a hard time and a trivial conversation about what require really is.
これらの違いは、ファイルが存在するかどうかによってエラーを発生させるかどうか。読み込み対象のファイルが存在しない場合、include
ではエラーは発生しないが require
では発生する。
2. 検証
存在するファイルと存在しないファイルを include
と require
で読み込むために次のようなフォームを用意した。
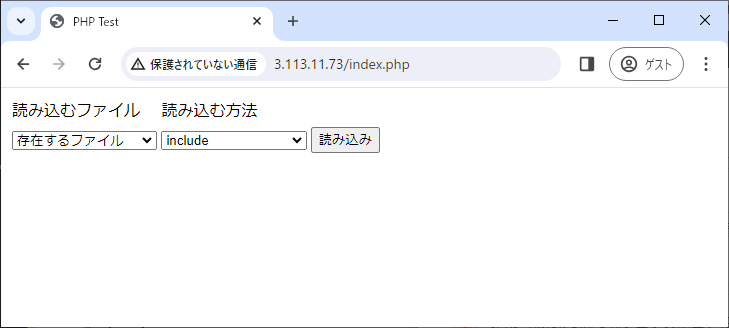
以下はそのコード:
<form method="POST"> <table> <tr> <td>読み込むファイル</td> <td>読み込む方法</td> </tr> <tr> <td> <select name="fileType" style="width: 20vw;"> <option value="existingFile">存在するファイル</option> <option value="nonexistentFile">存在しないファイル</option> </select> </td> <td> <select name="readType" style="width: 20vw;"> <option value="include">include</option> <option value="include_once">include_once</option> <option value="require">require</option> <option value="require_once">require_once</option> </select> </td> <td> <input type="submit" value="読み込み"/> </td> </tr> </table> </form>
POST メソッドは次のように処理する:
<?php if ($_SERVER["REQUEST_METHOD"] == "POST") { $file_type = ''; // existing_file.php は実際に同階層に用意しておく if ($_POST['fileType'] == 'existingFile') { $file_type = '存在するファイル'; $file_name = 'existing_file.php'; } else { $file_type = '存在しないファイル'; $file_name = '_.php'; } $read_type = $_POST['readType']; switch($read_type) { case 'include': include $file_name; break; case 'include_once': include_once $file_name; break; case 'require': require $file_name; break; case 'require_once': require_once $file_name; break; } echo "$file_type を $read_type で読み込みました"; }
existing_file.php
は次の内容で作成する:
<?php echo basename(__FILE__) . 'が読み込まれました<br>'; ?>
- 存在するファイルを
include
で読み込んだ時の挙動
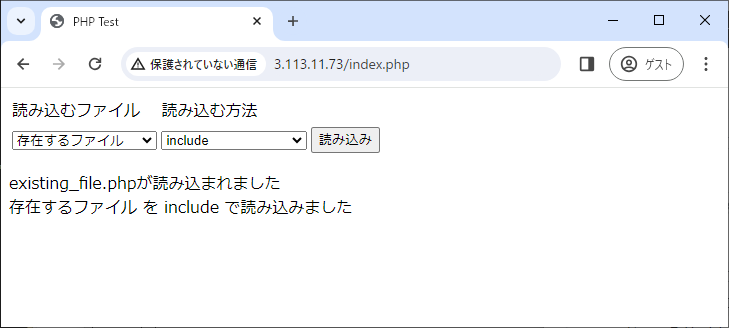
- 存在しないファイルを
include
で読み込んだ時の挙動
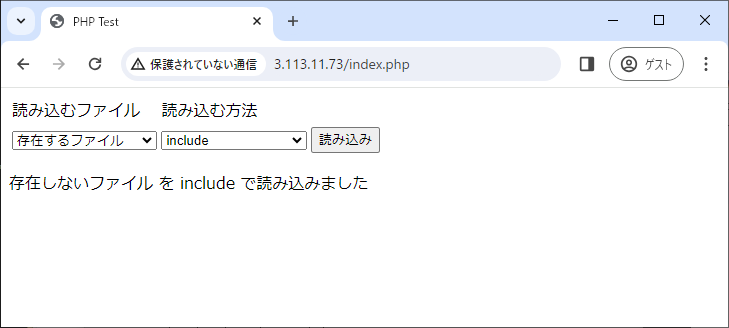
このとき /var/log/php-fpm/www-error.log
には次の警告ログが出力された:
[05-Jan-2024 13:06:50 UTC] PHP Warning: include(_.php): Failed to open stream: No such file or directory in /var/www/html/index.php on line 56 [05-Jan-2024 13:06:50 UTC] PHP Warning: include(): Failed opening '_.php' for inclusion (include_path='.:/usr/share/pear:/usr/share/php') in /var/www/html/index.php on line 56
- 存在しないファイルを
require
で読み込んだ時の挙動
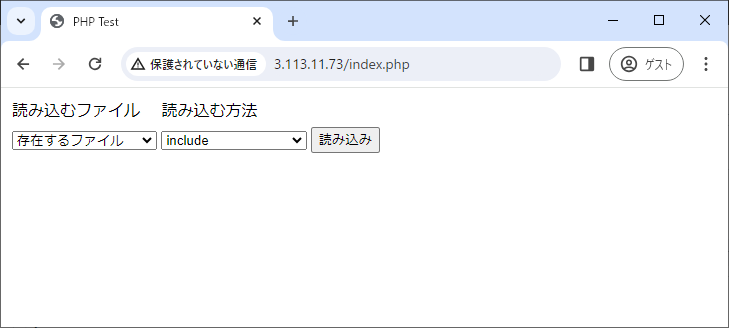
このとき /var/log/php-fpm/www-error.log
には次のエラーログが出力された:
[05-Jan-2024 13:12:36 UTC] PHP Warning: require(_.php): Failed to open stream: No such file or directory in /var/www/html/index.php on line 62 [05-Jan-2024 13:12:36 UTC] PHP Fatal error: Uncaught Error: Failed opening required '_.php' (include_path='.:/usr/share/pear:/usr/share/php') in /var/www/html/index.php:62